See Groq's STT in action with our realtime transcription playground
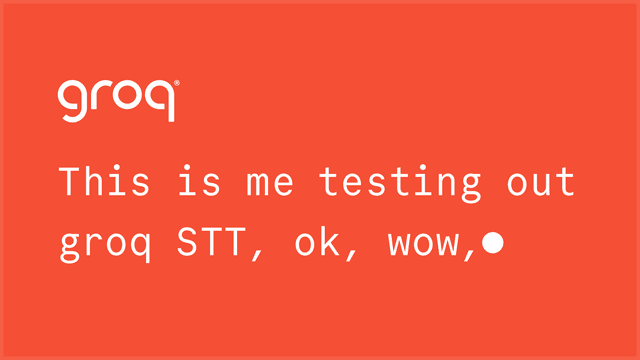
Overview
Groq provides low-latency AI inference with deterministic results and automatic speech recognition. LiveKit's Groq integration provides both STT and LLM functionality through an OpenAI-compatible interface. Use Groq and the Agents framework to build AI voice assistants that are realistic and predictable with accurate transcriptions.
This guide walks you through the steps to build a live transcription application that uses LiveKit's Agents Framework and the Groq STT service. For a demonstration of the following application, see the LiveKit and Groq transcription app.
If you're looking to build an AI voice assistant with Groq, check out our Voice Agent Quickstart guide and use the Groq integration as your STT and/or LLM provider.
Prerequisites
- Groq API Key
- Python 3.9-3.12 or Node 20.17.0 or higher
Instructions
Setup a LiveKit account and install the CLI
Create an account or sign in to your LiveKit Cloud account.
Install the LiveKit CLI and authenticate using
lk cloud auth
— (Optional).
The LiveKit CLI utility lk
is a convenient way to setup and configure applications and manage your LiveKit services, but installing it isn't required.
Bootstrap an agent from template
Clone a starter template for your preferred language using the CLI:
lk app create \--template-url https://github.com/livekit-examples/transcription-groq-pythonIf you aren't using the LiveKit CLI, clone the repository yourself:
git clone https://github.com/livekit-examples/transcription-groq-pythonEnter your Groq API Key when prompted or manually add your environment variables:
GROQ_API_KEY=<your-groq-api-key>LIVEKIT_API_KEY=<your-livekit-api-key>LIVEKIT_API_SECRET=<your-livekit-api-secret>LIVEKIT_URL=<your-livekit-url>Install dependencies and start your agent:
cd <agent_dir>python3 -m venv venvsource venv/bin/activatepython3 -m pip install -r requirements.txtpython3 main.py dev
For more details on using the STT module to perform transcription outside of the context of a voice pipeline or multimodal agent, see the transcriptions documentation.
Create a minimal frontend with Next.js
Clone the Transcription Frontend Next.js app starter template using the CLI:
lk app create --template transcription-frontendIf you aren't using the LiveKit CLI, clone the repository yourself:
git clone https://github.com/livekit-examples/transcription-frontendEnter your environment variables:
LIVEKIT_API_KEY=<your-livekit-api-key>LIVEKIT_API_SECRET=<your-livekit-api-secret>NEXT_PUBLIC_LIVEKIT_URL=<your-livekit-url>Install dependencies and start your frontend application:
cd <frontend_dir>pnpm installpnpm dev
Launch your app and talk to your agent
- Visit your locally-running application (by default, http://localhost:3000).
- Select Start voice transcription and begin speaking.
Quick reference
Environment variables
GROQ_API_KEY=<your-groq-api-key>
STT
LiveKit's Groq integration provides an OpenAI compatible speech-to-text (STT) interface. This can be used as the first stage in a VoicePipelineAgent
or as a standalone transcription service as documented above. For a complete reference of all available parameters, see the plugin reference.
Usage
from livekit.plugins.openai import sttgroq_stt = stt.STT.with_groq(model="whisper-large-v3-turbo",language="en",)
Parameters
ID of the model to use for inference. See supported models.
Whether or not language should be detected from the audio stream. Not every model
supports language detection. See supported models.
LLM
LiveKit's Groq integration also provides an OpenAI compatible LLM interface. This can be used in a VoicePipelineAgent
. For a complete reference of all available parameters, see the plugin reference.
Usage
from livekit.plugins.openai import llmgroq_llm = llm.LLM.with_groq(model="llama3-8b-8192",temperature=0.8,)
Parameters
ID of the model to use for inference. For a complete list, see supported models.
A measure of randomness of completions. A lower temperature is more deterministic. To learn more, see chat completions.